uploadFolder()
Uploads a group of files to Irys in a single transaction.
See:
Funding
Uploads of less than 100 KiB are are free. For larger uploads, you'll need to fund your account first.
NodeJS
Parameters
https://gateway.irys.xyz/:manifestId
.Returns
{
id, // Transaction ID (used to download the data)
timestamp, // Timestamp (UNIX milliseconds) of when the transaction was created and verified
version, // The version of this JSON file, currently 1.0.0
public, // Public key of the bundler node used
signature, // A signed deep hash of the JSON receipt
deadlineHeight, // The block number by which the transaction must be finalized
block, // Deprecated
validatorSignatures, // Deprecated
verify, // An async function used to verify the receipt at any time
}
Example
Use a token-specific version of getIrysUploader()
to connect to an Irys Bundler before uploading. Choose one from here.
const irys = await getIrysUploader();
// Upload an entire folder
const folderToUpload = "./my-images/"; // Path to folder
try {
const response = await irys.uploadFolder("./" + folderToUpload, {
indexFile: "", // Optional index file (file the user will load when accessing the manifest)
batchSize: 50, // Number of items to upload at once
keepDeleted: false, // Whether to keep now deleted items from previous uploads
});
console.log(`Files uploaded. Manifest ID ${response.id}`);
} catch (e) {
console.log("Error uploading file ", e);
}
Browser
Pass an array of File
objects.
Parameters
Returns
{
id, // Manifest ID
timestamp, // Timestamp (UNIX milliseconds) of when the transaction was created and verified
version, // The version of this JSON file, currently 1.0.0
public, // Public key of the bundler node used
signature, // A signed deep hash of the JSON receipt
deadlineHeight, // The block number by which the transaction must be finalized
block, // Deprecated
validatorSignatures, // Deprecated
verify, // An async function used to verify the receipt at any time
}
Example
const irysUploader = await getIrysUploader();
const files: File[] = [];
const tags: { name: string; value: string }[][] = [];
// Set files and tags in your UI
// Convert to TaggedFile objects
const taggedFiles = files.map((f: TaggedFile, i: number) => {
f.tags = tags[i];
return f;
});
// Optional parameters
const uploadOptions = {
indexFileRelPath: "./index.html",
manifestTags: myTags,
throwawayKey: myKey,
seperateManifestTx: true,
};
const response = await irysUploader.uploadFolder(taggedFiles, uploadOptions);
Returns
A JSON object containing the following values. A receipt is also generated which can be retrieved using irys.utils.getReceipt(response.id)
{
id, // Transaction ID
manifestId, // Manifest ID
manifest, // The manifest
txs, // An array of DataItems, one for each entry in the bundle
timestamp, // Timestamp (UNIX milliseconds) of when the transaction was created and verified
version, // The version of this JSON file, currently 1.0.0
public, // Public key of the bundler node used
signature, // A signed deep hash of the JSON receipt
deadlineHeight, // The block number by which the transaction must be finalized
block, // Deprecated
validatorSignatures, // Deprecated
verify, // An async function used to verify the receipt at any time
}
Downloading
Files uploaded via uploadFolder()
can be retrieved in one of two ways.
- Creating a URL with the format
https://gateway.irys.xyz/:manifestId/:originalFileName
. - Creating a URL using the transaction ID of each individual file uploaded with the format
https://gateway.irys.xyz/:transactonId
After a successful folder upload, two files are written to your local project directory [folder_name].csv
and [folder_name].json
. The example below highlights a folder called with a total of 5 files in it. The transaction ID for each file can be used to retrieve the uploaded data by forming a URL with the format https://gateway.irys.xyz]/:transactionId
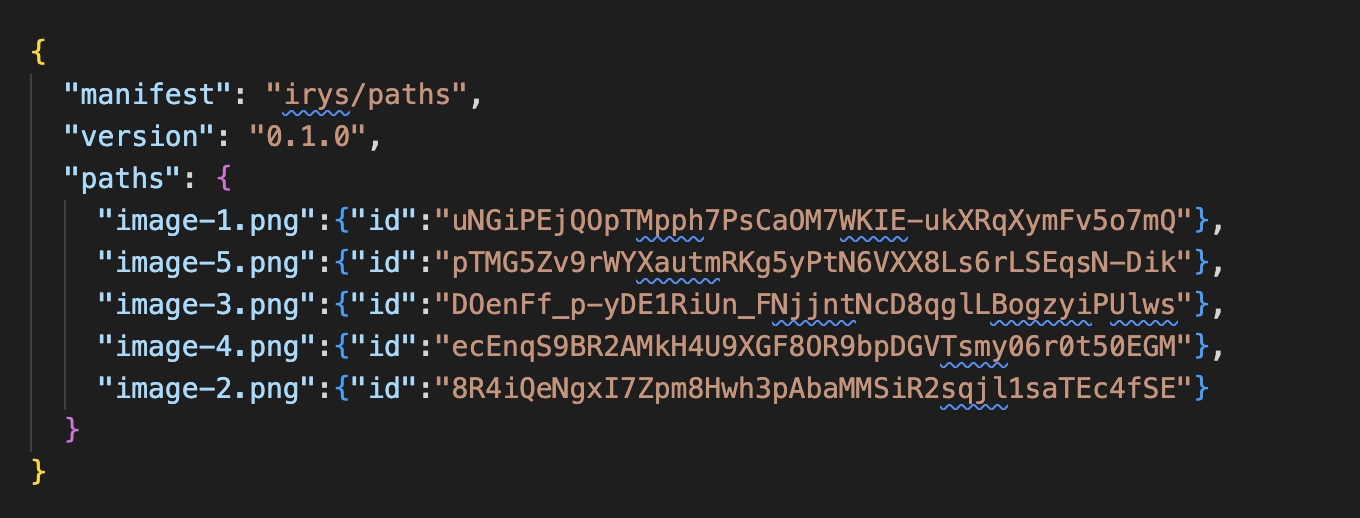